The RTC keeps the date and time arranged in it's memory as shown below:

Slave Receiver Mode (Write Mode)
Data Write Cycle
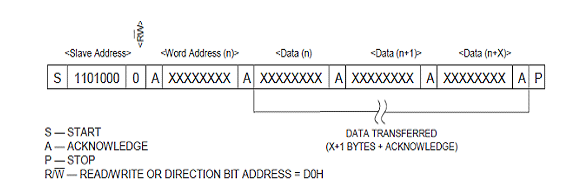
Slave Transmitter Mode (Read Mode)
Data Read Cycle
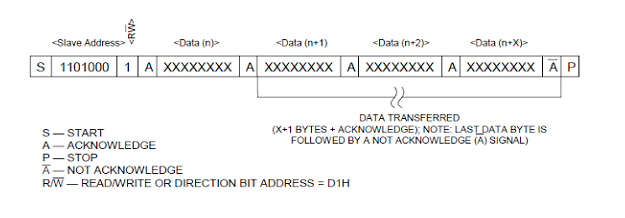
TWI Status Register – TWSR

Bits 7..3 – TWS: TWI Status
Bit 2 – Res: Reserved Bit
Bits 1..0 – TWPS: TWI Prescaler Bits:
TWI Data Register – TWDR

Bits 7..0 – TWD: TWI Data Register
/* Name : main.c * Purpose : Source code for RTC interface with ATMEGA16. * Author : Gemicates * Date : 2017-09-07 * Website : www.gemicates.org * Revision : None */ #ifndef F_CPU #define F_CPU 8000000UL // 8 MHz clock speed #endif #include <avr/io.h> #include <util/delay.h> #define UCSRA #define UCSRB #define UCSRC #define UDR #define UBRRL void init_i2c() { TWSR = 0X00; TWBR = 0X47; TWCR = (1<<TWEN); } unsigned char read_i2c() { TWCR = (1<<TWINT)|(1<<TWEN); while(!(TWCR & (1<<TWINT))); return TWDR; } void write_i2c(unsigned char ch) { TWDR = ch; TWCR = (1<<TWINT)|(1<<TWEN)|(1<<TWEA); while(!(TWCR&(1<<TWINT))); } void start() { TWCR = (1<<TWINT)|(1<<TWSTA)|(1<<TWEN); while((TWCR &(1<<TWINT))==0); } void stop() { TWCR = (1<<TWINT)|(1<<TWEN)|(1<<TWSTO); _delay_ms(1); } void rtc_write(char dev_addr,char dev_loc,char dev_data) { start(); write_i2c(dev_addr); write_i2c(dev_loc); write_i2c(dev_data); stop(); _delay_ms(10); } unsigned char rtc_read(char dev_addr,char dev_loc) { char ch; start(); write_i2c(dev_addr); write_i2c(dev_loc); start(); write_i2c(dev_addr|0x01); ch = read_i2c(); stop(); return ch; } void tx( unsigned char data ) { while ( !(UCSRA (1<<UDRE)) ); // wait until UDR is empty UDR data; } char rx() { while ( !(UCSRA (1<<RXC)) ); // wait until UDR is empty return UDR; } void init_serial() { UCSRB (1<<RXEN)|(1<<TXEN); UCSRC (1<<UCSZ1)|(1<<UCSZ0); UBRRL 51; } void str_serial(char *str) { while(*str) { tx(*str++); _delay_ms(1); } } void disp_time_date() { char ch; tx(254); tx(128); str_serial("Time: "); ch = rtc_read(0xd0 , 0x02); tx(ch/16+48); tx(ch%16+48); tx('-'); ch = rtc_read(0xd0 , 0x01); tx(ch/16+48); tx(ch%16+48); tx('-'); ch = rtc_read(0xd0 , 0x00); tx(ch/16+48); tx(ch%16+48); tx(254); tx(192); str_serial("Date: "); ch = rtc_read(0xd0 , 0x04); tx(ch/16+48); tx(ch%16+48); tx('-'); ch = rtc_read(0xd0 , 0x05); tx(ch/16+48); tx(ch%16+48); tx('-'); ch = rtc_read(0xd0 , 0x06); tx(ch/16+48); tx(ch%16+48); } int main() { DDRC =0XFF; DDRD =0XFF; init_i2c(); init_serial(); rtc_write(0xd0,0x00,0x00); rtc_write(0xd0,0x01,0x27); rtc_write(0xd0,0x02,0x08); rtc_write(0xd0,0x04,0x08); rtc_write(0xd0,0x05,0x09); rtc_write(0xd0,0x06,0x13); _delay_ms(10000); while(1) { disp_time_date(); } }